Spring Boot AOP The application is generally developed with multiple layers. A typical Java application has the following layers:
- Web Layer: It exposes the services using the REST or web application.
- Business Layer: It implements the business logic of an application.
- Data Layer: It implements the persistence logic of the application.
The responsibility of each layer is different, but there are a few common aspects that apply to all layers are Logging, Security, validation, caching, etc. These common aspects are called cross-cutting concerns. If we implement these concerns in each layer separately, the code becomes more difficult to maintain. To overcome this problem, Aspect-Oriented Programming (AOP) provides a solution to implement cross-cutting concerns.
- Implement the cross-cutting concern as an aspect.
- Define pointcuts to indicate where the aspect has to be applied.
It ensures that the cross-cutting concerns are defined in one cohesive code component. AOP AOP (Aspect-Oriented Programming) is a programming pattern that increases modularity by allowing the separation of the cross-cutting concern. These cross-cutting concerns are different from the main business logic. We can add additional behavior to existing code without modification of the code itself. Spring's AOP framework helps us to implement these cross-cutting concerns. Using AOP, we define common functionality in one place. We are free to define how and where this functionality is applied without modifying the class to which we are applying the new feature. The cross-cutting concern can now be modularized into special classes, called aspect. There are two benefits of aspects:
- First, the logic for each concern is now in one place instead of scattered all over the codebase.
- Second, the business modules only contain code for their primary concern. The secondary concern has been moved to the aspect.
The aspects have the responsibility that is to be implemented, called advice. We can implement an aspect's functionality into a program at one or more join points. Benefits of AOP
- It is implemented in pure Java.
- There is no requirement for a special compilation process.
- It supports only method execution Join points.
- Only run time weaving is available.
- Two types of AOP proxy is available: JDK dynamic proxy and CGLIB proxy.
Cross-cutting concern The cross-cutting concern is a concern that we want to implement in multiple places in an application. It affects the entire application. AOP Terminology
- Aspect: An aspect is a module that encapsulates advice and pointcuts and provides cross-cutting An application can have any number of aspects. We can implement an aspect using regular class annotated with @Aspect annotation.
- Pointcut: A pointcut is an expression that selects one or more join points where advice is executed. We can define pointcuts using expressions or patterns. It uses different kinds of expressions that matched with the join points. In Spring Framework, AspectJ pointcut expression language is used.
- Join point: A join point is a point in the application where we apply an AOP aspect. Or it is a specific execution instance of an advice. In AOP, join point can be a method execution, exception handling, changing object variable value, etc.
- Advice: The advice is an action that we take either before or after the method execution. The action is a piece of code that invokes during the program execution. There are five types of advices in the Spring AOP framework: before, after, after-returning, after-throwing, and around advice. Advices are taken for a particular join point. We will discuss these advices further in this section.
- Target object: An object on which advices are applied, is called the target object. Target objects are always a proxied It means a subclass is created at run time in which the target method is overridden, and advices are included based on their configuration.
- Weaving: It is a process of linking aspects with other application types. We can perform weaving at run time, load time, and compile time.
Proxy: It is an object that is created after applying advice to a target object is called proxy. The Spring AOP implements the JDK dynamic proxy to create the proxy classes with target classes and advice invocations. These are called AOP proxy classes. AOP vs. OOP The differences between AOP and OOP are as follows:
AOP |
OOP |
Aspect: A code unit that encapsulates pointcuts, advices, and attributes. |
Class: A code unit that encapsulates methods and attributes. |
Pointcut: It defines the set of entry points in which advice is executed. |
Method signature: It defines the entry points for the execution of method bodies. |
Advice: It is an implementation of cross-cutting concerns. |
Method bodies: It is an implementation of the business logic concerns. |
Waver: It constructs code (source or object) with advice. |
Compiler: It converts source code to object code. |
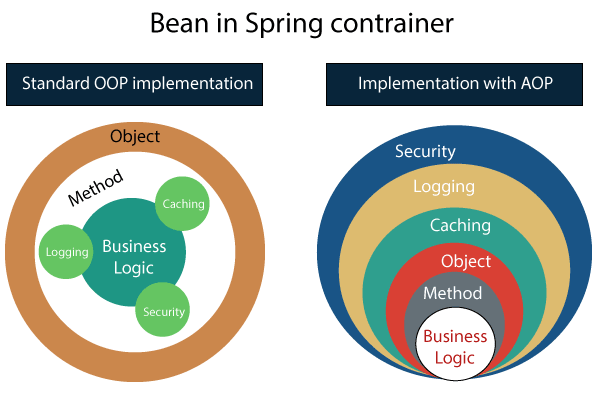 Spring AOP vs. AspectJ The differences between AOP and OOP are as follows:
Spring AOP |
AspectJ |
There is a need for a separate compilation process. |
It requires the AspectJ compiler. |
It supports only method execution pointcuts. |
It supports all pointcuts. |
It can be implemented on beans managed by Spring Container. |
It can be implemented on all domain objects. |
It supports only method level weaving. |
It can wave fields, methods, constructors, static initializers, final class, etc. |
Types of AOP Advices There are five types of AOP advices are as follows:
- Before Advice
- After Advice
- Around Advice
- After Throwing
- After Returning
Before Advice: An advice that executes before a join point, is called before advice. We use @Before annotation to mark an advice as Before advice. After Advice: An advice that executes after a join point, is called after advice. We use @After annotation to mark an advice as After advice. Around Advice: An advice that executes before and after of a join point, is called around advice. After Throwing Advice: An advice that executes when a join point throws an exception. After Returning Advice: An advice that executes when a method executes successfully. Before implementing the AOP in an application, we are required to add Spring AOP dependency in the pom.xml file. Spring Boot Starter AOP Spring Boot Starter AOP is a dependency that provides Spring AOP and AspectJ. Where AOP provides basic AOP capabilities while the AspectJ provides a complete AOP framework.
In the next section, we will implement the different advices in the application.
|